Understanding Fabs() In C/C++: Syntax, Usage, & Examples
Is understanding the intricacies of absolute values in programming crucial for writing robust and efficient code? Absolutely. Functions like `fabs()` and `std::abs()` are fundamental tools in C++ and C, providing the means to calculate the magnitude of a number, irrespective of its sign. These seemingly simple functions unlock a myriad of possibilities, impacting everything from scientific computations to data processing.
The world of programming often demands the ability to work with numerical values, but sometimes, the sign of a number is irrelevant. Consider scenarios where you need to measure the distance between two points, determine the error in a calculation, or process data where only the magnitude is significant. This is where the concept of absolute value comes into play. The `fabs()` function and its counterparts are designed to address this need, returning the non-negative value of a given number.
Let's delve into the specifics of the `fabs()` function and its role in C++ and C programming. Understanding its syntax, parameters, return values, and nuances is essential for any programmer looking to write clean, efficient, and error-free code.
At its core, the `fabs()` function is designed to return the absolute value of a floating-point number. It's a fundamental mathematical operation that finds extensive application in various domains, including physics simulations, financial modeling, and signal processing. The core function returns the magnitude of a number, effectively stripping away its sign. The syntax is straightforward, and its use is simple.
Function | Description | Syntax | Parameters | Return Value | Header File | Compiler Support |
---|---|---|---|---|---|---|
fabs() | Returns the absolute value of a floating-point number. | double fabs(double x); float fabsf(float x); long double fabsl(long double x); |
| The absolute value of x . | #include | All major C and C++ compilers. Support for specific overloads (e.g., fabsl ) may vary depending on the C standard and compiler. |
In C++, the standard library provides a similar function, `std::abs()`, but it's overloaded to handle both integer and floating-point types. `std::fabs()`, on the other hand, is primarily concerned with floating-point types (pre-C++11). The `fabs()` function in C++ returns the absolute value of the argument. It is defined within the `` header file.
The C function `::abs()`, often present for legacy reasons, is specifically designed for integer types. It's essential to choose the correct function based on the data type you're working with to ensure accurate results.
The usage of `fabs()` is generally straightforward. Include the `` header file, and then you can call the function with your floating-point number as an argument. The function then returns the absolute value of that number.
Here's an example demonstrating its usage in C++:
#include #include // Required for fabs()int main() { double number = -3.14; double absoluteValue = fabs(number); std::cout << "The absolute value of " << number << " is: " << absoluteValue << std::endl; return 0;}
This code snippet demonstrates how to include the necessary header file and how to call the function. The output will be:
The absolute value of -3.14 is: 3.14
The `fabs()` function is a fundamental building block in numerical programming, enabling calculations where the sign of a number is not important.
In essence, the `fabs()` function empowers programmers to handle floating-point numbers effectively by providing their magnitudes.
When dealing with different floating-point types, consider the overloaded versions: `fabsf()` for `float` and `fabsl()` for `long double`. This ensures the correct precision and type safety in your code. Since C23, there are even more specialized versions available for decimal floating-point types such as `fabsd32()`, `fabsd64()` and `fabsd128()`, allowing for greater flexibility.
Understanding the differences between these functions and `std::abs()` is important, as `std::abs()` offers broader support but might lead to subtle differences in behavior depending on the context.
In more complex scenarios, such as those involving complex numbers, specialized functions like `cabsf()`, `cabs()`, and `cabsl()` are used. However, the core principle of finding the magnitude of a number remains the same.
Error handling in relation to `fabs()` is generally not a major concern because it's a well-defined mathematical function. There are no specific error conditions that `fabs()` directly handles. However, it's crucial to be mindful of potential issues like undefined behavior resulting from passing NaN (Not a Number) values as arguments.
Here's a quick summary of the key points for using `fabs()` effectively:
- Include the `` header file.
- Use `fabs()` for `double` values, `fabsf()` for `float` values, and `fabsl()` for `long double` values.
- Be aware of the differences compared to `std::abs()`, especially when working with integers.
- Use appropriate functions for complex numbers if needed (e.g., `cabs()`).
The utility of `fabs()` extends far beyond simple calculations.
In computer graphics, it helps calculate distances and perform transformations.
In various scientific fields, the function provides a standardized way to determine the magnitude of data.
The adaptability of `fabs()` makes it a vital function for a wide variety of computational tasks.
The implementation of `fabs()` is typically highly optimized by the compiler for the target platform. This optimization ensures that calculating the absolute value is performed efficiently, minimizing any performance impact on your code.
The function has a time complexity of O(1), indicating a constant time operation that doesn't depend on the size of the input. The auxiliary space usage is also constant, as the function doesn't require significant additional memory to perform its calculations.
The choice between `fabs()` and other absolute value functions depends on the context of your program. When working with floating-point types, `fabs()` is the appropriate choice. If the input data type is an integer, use `std::abs()`. The use of the correct function ensures both accuracy and efficiency in your programs.
The absolute value functions are a cornerstone of many programming tasks.
By mastering the use of `fabs()`, programmers can improve the quality and reliability of their code.
The availability and standardization of these functions across different compilers and platforms make them invaluable tools for any programmer seeking to build robust and reliable software.
In conclusion, the `fabs()` function and its related functions are indispensable components of the C and C++ programming languages. These functions provide a simple yet powerful tool for calculating the absolute value of floating-point numbers, making them essential for various computational tasks. Understanding the syntax, parameters, return values, and implications of using these functions will enable programmers to create precise, efficient, and versatile code.
The importance of these tools extends far beyond introductory examples. They are at the heart of many scientific, engineering, and financial calculations. Mastering these functions is therefore, a key element of programming.
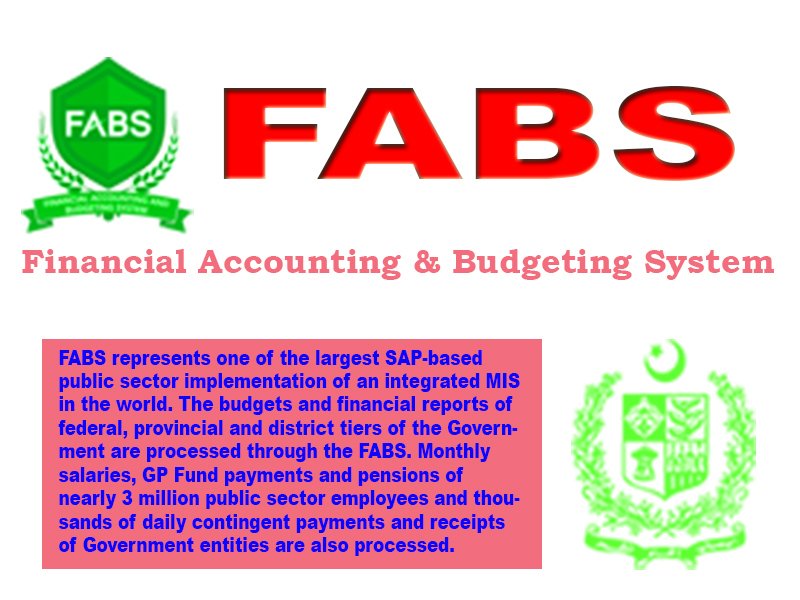


Detail Author:
- Name : Mona Torphy I
- Email : sadye.raynor@stamm.org
- Birthdate : 1997-02-09
- Address : 475 Hamill Ridge Port Ethelyn, WY 14912-8972
- Phone : +1-256-427-7512
- Company : Schmitt and Sons
- Job : Licensed Practical Nurse
- Bio : Iusto asperiores molestiae sint soluta. Sed sapiente velit pariatur cum sed provident. Optio ut amet dolore aut quod quidem.